一、前言
定时器,项目中遇到的应该不多,就比如我本人,到至今,在项目中使用到定时器也就三两次,在springboot还未出来之前,使用定时器估计是很难受的,虽然我没怎么用过,因为我刚开始工作的时候springboot出来了,然后真香,哈哈哈哈哈……
二、java上古时代的定时器实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80
| package com.example.demo; import java.time.LocalDateTime; import java.util.*; import java.util.concurrent.Executors; import java.util.concurrent.ScheduledExecutorService; import java.util.concurrent.TimeUnit; public class AllController { public static void main(String[] args) { runnable(); timerTask(); thread(); }
public static void runnable() { Runnable runnable = new Runnable() { public void run() { System.out.println("HelloWorld!!!" + LocalDateTime.now()); } }; ScheduledExecutorService service = Executors.newSingleThreadScheduledExecutor(); service.scheduleAtFixedRate(runnable, 1, 5, TimeUnit.SECONDS); }
public static void timerTask() { TimerTask task = new TimerTask() { @Override public void run() { System.out.println("HelloWorld!!!" + LocalDateTime.now()); } }; Timer timer = new Timer(); long wait = 5000; long wait1 = 1 * 1000; timer.scheduleAtFixedRate(task, wait, wait1); }
public static void thread() { final long timeInterval = 1000; Runnable runnable = new Runnable() { public void run() { while (true) { System.out.println("HelloWorld!!!" + LocalDateTime.now()); try { Thread.sleep(timeInterval); } catch (InterruptedException e) { e.printStackTrace(); } } } }; Thread thread = new Thread(runnable); thread.start(); } }
|
三、现代的springboot下实现
第一种
1、在启动类上添加注解@EnableScheduling开启定时器
1 2 3 4 5 6 7 8
| @SpringBootApplication @EnableScheduling public class DemoApplication { public static void main(String[] args) { SpringApplication.run(DemoApplication.class, args); } }
|
2、给要定时执行的方法上添加注解@Scheduled(cron = “0 0 0 * * * “)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| package com.example.demo; import org.springframework.scheduling.annotation.Scheduled; import org.springframework.stereotype.Component; import java.time.LocalDateTime; @Component public class ScheduleTask1 { @Scheduled(cron = "0/2 * * * * *") public void runTimer() { System.out.println("HelloWorld!!!" + LocalDateTime.now()); } }
|
第二种
直接在定时器类上添加@Configuration、@EnableScheduling注解,标注这个类是配置文件,并开启定时开关;然后给要定时执行方法上添加@Scheduled(cron = “0 0 0 * * * “)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| package com.example.demo; import org.springframework.context.annotation.Configuration; import org.springframework.scheduling.annotation.EnableScheduling; import org.springframework.scheduling.annotation.Scheduled; import java.time.LocalDateTime; @Configuration @EnableScheduling public class ScheduleTask { @Scheduled(cron = "0/5 * * * * ?") public void tasks() { System.out.println("HelloWorld!!!" + LocalDateTime.now()); } }
|
四、附上cron表达式
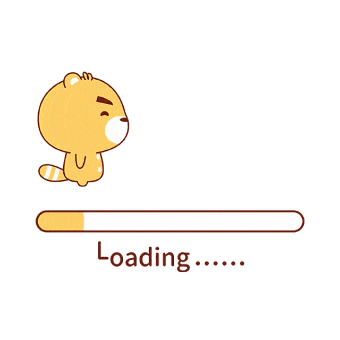
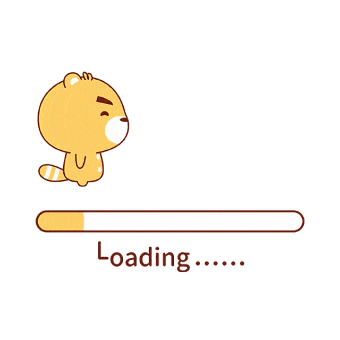
五、结语
暂时写到这里,有兴趣的可以自己测一下或者实现一下……